I add 3 buttons to customized case section.
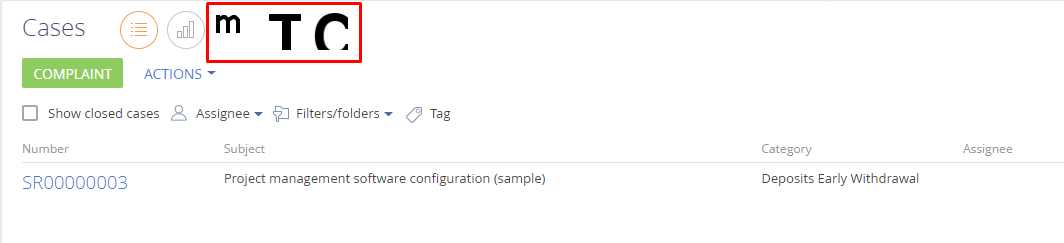
With this code:
define("CaseSection", [], function() {
return {
entitySchemaName: "Case",
details: /**SCHEMA_DETAILS*/{}/**SCHEMA_DETAILS*/,
attributes: {},
methods: {
getDefaultDataViews: function() {
var baseDataViews = this.callParent(arguments);
baseDataViews.GridDataViewMyCases = {
name: "GridDataViewMyCases",
caption: this.get("Resources.Strings.MyCasesButtonCaption"), // Section header
hint: this.get("Resources.Strings.MyCasesButtonCaption"), // Hint for button
icon: this.get("Resources.Images.MyCasesDataViewIcon") // Image for button
};
baseDataViews.GridDataViewTeamCases = {
name: "GridDataViewTeamCases",
caption: this.get("Resources.Strings.TeamCasesButtonCaption"), // Section header
hint: this.get("Resources.Strings.TeamCasesButtonCaption"), // Hint for button
icon: this.get("Resources.Images.TeamCasesDataViewIcon") // Image for button
};
baseDataViews.GridDataViewManager = {
name: "GridDataViewManager",
caption: this.get("Resources.Strings.ManagerButtonCaption"), // Section header
hint: this.get("Resources.Strings.ManagerButtonCaption"), // Hint for button
icon: this.get("Resources.Images.ManagerDataViewIcon") // Image for button
};
return baseDataViews;
},
loadActiveViewData: function() {
var activeViewName = this.getActiveViewName();
if (activeViewName === "GridDataViewMyCases") {
this.loadGridData();
}
else if (activeViewName === "GridDataViewTeamCases") {
this.loadGridData();
}
else if (activeViewName === "GridDataViewManager") {
this.loadGridData();
}
this.callParent(arguments);
},
loadGridDataView: function(loadData) {
var gridData = this.getGridData();
if (gridData && loadData) {
gridData.clear();
}
this.setViewFilter(this.get("ActiveViewName"));
this.reloadGridColumnsConfig(false);
this.reloadSummaryModule();
this.callParent(arguments);
},
loadGridDataViewMyCases: function(loadData) { // "load" + DataView.name
this.loadGridDataView(loadData);
},
loadGridDataViewTeamCases: function(loadData) { // "load" + DataView.name
this.loadGridDataView(loadData);
},
loadGridDataViewManager: function(loadData) { // "load" + DataView.name
this.loadGridDataView(loadData);
},
setActiveView: function(activeViewName) {
this.callParent(arguments);
if (activeViewName === "GridDataViewMyCases") {
this.set("IsGridDataViewVisible", true);
}
else if (activeViewName === "GridDataViewTeamCases") {
this.set("IsGridDataViewVisible", true);
}
else if (activeViewName === "GridDataViewManager") {
this.set("IsGridDataViewVisible", true);
}
},
setViewFilter: function(activeViewName) { // Add filter for your "DataView"
var sectionFilters = this.get("SectionFilters");
if (activeViewName === "GridDataViewMyCases") {
sectionFilters.add("FilterB2BType", this.Terrasoft.createColumnFilterWithParameter(
this.Terrasoft.ComparisonType.NOT_EQUAL, "Status.Id", "3E7F420C-F46B-1410-FC9A-0050BA5D6C38"));
}
else if (activeViewName === "GridDataViewTeamCases") {
sectionFilters.add("FilterB2BType", this.Terrasoft.createColumnFilterWithParameter(
this.Terrasoft.ComparisonType.NOT_EQUAL, "UsrStatus", "Closed"));
}
else if (activeViewName === "GridDataViewManager") {
sectionFilters.add("FilterB2BType", this.Terrasoft.createColumnFilterWithParameter(
this.Terrasoft.ComparisonType.NOT_EQUAL, "UsrStatus", "Closed"));
} else {
sectionFilters.removeByKey("FilterB2BType");
}
}
}
};
});
I defined all strings (MyCasesButtonCaption, MyCasesDataViewIcon, TeamCasesButtonCaption, TeamCasesDataViewIcon, ManagerButtonCaption, ManagerDataViewIcon) on case section structure.
But when I select one of them, the button disappears:
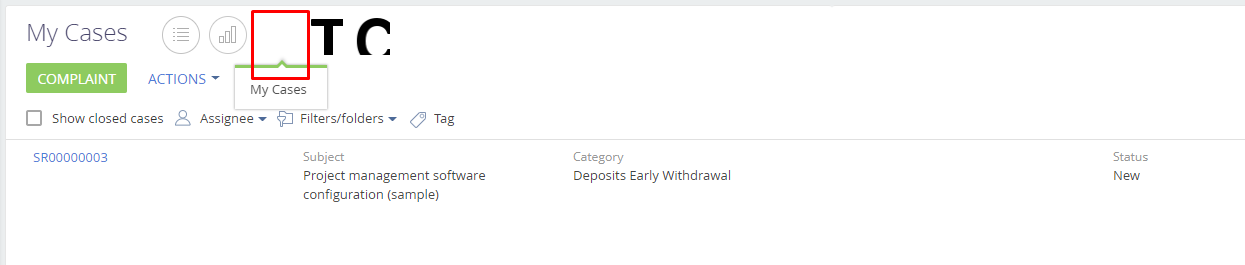
Can you help me?
Regards